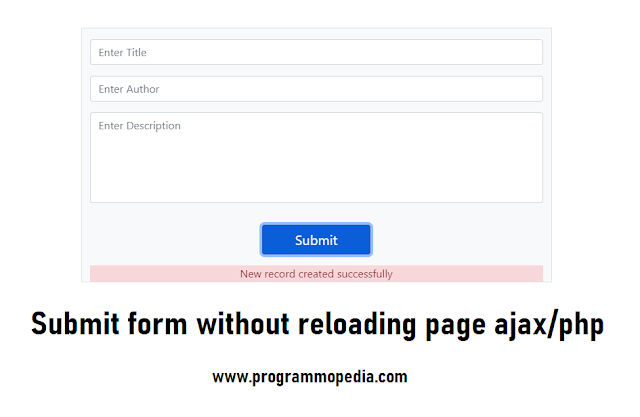
What Is AJAX?
AJAX is an acronym for Asynchronous JavaScript And XML. It's not a
programming language but a programming technique. It is a technology that allows web pages to fetch and transmit data without reloading or refreshing the entire webpage. It updates the content asynchronously(in the background)
without letting the user know about any transmission of data to the server.
Now let's use this information to
submit form without reloading page in ajax and php.
How to submit form without reloading page ajax/php?
Let's first divide the complete concept of the article into steps.
- Create a table in your MYSQL database using SQL query.
- Create a simple HTML form design using Bootstrap 5.
- Write Jquery AJAX script to transmit the data asynchronously to the pure PHP page through the POST method.
- Write PHP script to insert data to the database and then send the response back to the AJAX script.
Now let's explain the above-mentioned steps one by one.
How to create table in database using SQL query?
- Create a table "books" in the database "library".
- Create 4 colmns, id, title, author, and description in the table.
- Make the first column a primary key and also use the auto-increment keyword.
- Set the domain of title and author to varchar and set their length value to 200.
- Set the length value of the description column to 2000 and its domain will be text.
CREATE TABLE `library`.`books`( `id` INT(100) NOT NULL AUTO_INCREMENT ,`title` VARCHAR(200) NOT NULL ,`author` VARCHAR(200) NOT NULL ,`description` TEXT NOT NULL ,PRIMARY KEY (`id`)) ENGINE = InnoDB;
In this way, we can create a database table with four fields. The id field value is auto-incremented and unique. That's why it is used as a
primary key for this table. The other three fields will store string data.
How to create an HTML form using bootstrap?
- To use bootstrap, add bootstrap CDN in the head section of your code. Or copy the starter template available on getbootstrap.com. It has everything we need to create a design using bootstrap.
- Create a simple bootstrap one column Grid on your webpage. You can use the justify-content-center and text center classes to align the content to center on the webpage.
- Create an Html form with id "form". We will use this id later in jquery to empty the text fields after the form submission. Don't bother about that now.
- Create two text fields with ids "title" and "author" respectively.
- Create one text area and set its id attribute to "desc".
- Create bootstrap alert div. Here we will display alert messages through Jquery.
<div class="row justify-content-center my-5 "><div class="col-6 text-center bg-light border"><form id="form"><div class="form-group my-3"><input type="text" class="form-control" placeholder="Enter Title" id="title" ></div><div class="form-group my-3"><input type="text" class="form-control" placeholder="Enter Author" id="author"></div><div class="form-group my-3"><textarea class="form-control" rows="5" id="desc" placeholder="Enter Description" style="resize: none;"></textarea></div><button type="submit" id="submit" name="post" class="btn btn-primary my-3 fs-5 px-5">Submit</button></form><div id="alert" class="alert-danger"> </div></div></div>
How to submit a form asynchronously using ajax?
- Use Jquery on() method to attach a click event to our submit button selected using id selector.
- Disable the default behavior of submit button to avoid page reload using the preventdefault() method.
- Store the values of input fields in variables using the val() method. Select the input fields using the id selector.
- Send this data to the "SubmitForm.inc.php" file using the ajax() method through the POST request.
- Write a function on the success of ajax request and display the message or response returned by the PHP page inside alert div using the html() method.
- Reset the form input fields using the trigger() method.
$(document).ready(function() {$("#submit").on("click", function(e) {e.preventDefault();var title = $("#title").val();var author = $("#author").val();var desc = $("#desc").val();$.ajax({url: "includes/SubmitForm.inc.php",type: "POST",data: {title: title,author: author,desc: desc},success: function($data) {$("#alert").html($data);$("#form").trigger("reset");}});});});
How to insert data in a database using PHP?
- Create the "SubmitForm.inc.php" file and open it to start PHP coding.
- First of all, connect to the database to execute the insert query.
$server_name = "localhost";$db_username = "root";$db_password = "";$db_name = "library";$conn = new mysqli($server_name, $db_username, $db_password, $db_name);if ($conn->connect_error) {die("Connection failed: " . $conn->connect_error);}
- Make sure the content of the form is not empty using the empty() function.
- Use the real_escape_string() function to escape special characters from the string and store the data of input fields in variables.
- Write and execute an insert query and check if it's executed successfully.
- Print success message on success and error message on the failure of the query using echo statements.
- These echo statements are the response or result that we will fetch using ajax in jquery code.
if (!empty($_POST['title']) && !empty($_POST['title']) && !empty($_POST['title'])) {$title = $conn->real_escape_string($_POST['title']);$author = $conn->real_escape_string($_POST['author']);$Desc = $conn->real_escape_string($_POST['desc']);$query = "INSERT INTO `books`( `title`, `author`, `description`)VALUES ('$title','$author','$Desc')";if ($conn->query($query) === TRUE) {echo "New record created successfully";} else {echo "Error: " . $query . "<br>" . $conn->error;}} else {echo "Warning! fill all input fields";}$conn->close();
Complete code to submit form without reloading ajax/php:
SubmitForm.php page containing HTML and Jquery code:
<!doctype html><html lang="en"><head><meta charset="utf-8"><meta name="viewport" content="width=device-width, initial-scale=1"><script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script><link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous"><title>Ajax php form</title></head><body><div class="row justify-content-center my-5 "><div class="col-6 text-center bg-light border"><form id="form"><div class="form-group my-3"><input type="text" class="form-control" placeholder="Enter Title" id="title"></div><div class="form-group my-3"><input type="text" class="form-control" placeholder="Enter Author" id="author"></div><div class="form-group my-3"><textarea class="form-control" rows="5" id="desc" placeholder="Enter Description" style="resize: none;"></textarea></div><button type="submit" id="submit" name="post" class="btn btn-primary my-3 fs-5 px-5">Submit</button></form><div id="alert" class="alert-danger"> </div></div></div><script>$(document).ready(function() {$("#submit").on("click", function(e) {e.preventDefault();var title = $("#title").val();var author = $("#author").val();var desc = $("#desc").val();$.ajax({url: "includes/SubmitForm.inc.php",type: "POST",data: {title: title,author: author,desc: desc},success: function($data) {$("#alert").html($data);$("#form").trigger("reset");}});});});</script></body></html>
SubmitFrom.inc.php page containing pure PHP code:
<?php$server_name = "localhost";$db_username = "root";$db_password = "";$db_name = "library";$conn = new mysqli($server_name, $db_username, $db_password, $db_name);if ($conn->connect_error) {die("Connection failed: " . $conn->connect_error);}if (!empty($_POST['title']) && !empty($_POST['title']) && !empty($_POST['title'])) {$title = $conn->real_escape_string($_POST['title']);$author = $conn->real_escape_string($_POST['author']);$Desc = $conn->real_escape_string($_POST['desc']);$query = "INSERT INTO `books`( `title`, `author`, `description`)VALUES ('$title','$author','$Desc')";if ($conn->query($query) === TRUE) {echo "New record created successfully";} else {echo "Error: " . $query . "<br>" . $conn->error;}} else {echo "Warning! fill all input fields";}$conn->close();
Output of the code:
Recommended:
Now it's time to conclude the article here. it was an introductory article to
AJAX where we learned how to submit form without reloading page using ajax and PHP. I hope you liked the shared information. Thanks for reading and supporting us.
0 Comments